Debounce vs throttle are two techniques used in JavaScript to control the frequency of executing a function, especially in scenarios involving frequent events like scrolling, resizing, or typing. While they serve a similar purpose, they have distinct characteristics and applications:
Debounce vs Throttle
Debounce:
Debouncing ensures that a function is executed only after a certain period of inactivity. It postpones the execution of the function until a specified interval of time has passed since the last invocation. If the function is called again within that interval, the timer resets, and the execution is further delayed. This helps to prevent rapid and frequent function invocations.
Debounce is useful in scenarios where you want to delay the execution of a function until a specific period of inactivity has occurred. For example, in an autocomplete search field, you can debounce the search function to avoid making API requests for every keystroke. Instead, the function is executed only when the user pauses typing for a certain duration.
Throttle:
Throttling limits the frequency of executing a function by enforcing a maximum execution rate. It ensures that the function is invoked at regular intervals, preventing it from being called more frequently than a predefined threshold. Throttling guarantees a fixed rate of function execution regardless of how frequently the function is triggered.
Throttle is beneficial when you want to control the frequency of function execution, especially for resource-intensive operations or events that trigger a high number of calls. For instance, in a scroll event handler, throttling can be used to limit the execution rate of a function that performs complex calculations or updates the UI. This way, the function is executed at regular intervals instead of firing on every scroll event.
In summary, debounce delays the execution of a function until a period of inactivity, while throttle limits the frequency of function execution to a predefined rate. Debounce is ideal when you want to wait for pauses before executing a function, while throttle is useful for ensuring a maximum rate of function execution. The choice between debounce and throttle depends on the specific requirements and behavior desired for your application.
JavaScript Debouncing
JavaScript debouncing is a methodology that ensures a function is triggered only after a designated time interval has elapsed without any further calls.
This approach proves invaluable in scenarios where a function is invoked frequently, such as when a user is actively entering text into an input field. Debouncing allows you to delay the execution of a particular action until the user has completed their input.
For instance, in situations where repeated clicks on the same button occur, debouncing ensures that the associated event is executed only after the last click, preventing multiple rapid executions.
const debounce = (fn,delay)=>{
let timeoutID;
return function(...args){
if(timeoutID){
clearTimeout(timeoutID)
}
timeoutID = setTimeout(()=>{
fn(...args);
}, delay);
}
}
document.getElementById("myid").addEventListner("click", debounce(e=>{
console.log('clicked');
},2000));
JavaScript Throttling
JavaScript throttling is similar to debouncing, but instead of waiting for a certain amount of time to pass before executing a function, it limits the number of times the function can be called within a specific period of time.
This is useful in situations where a function is called frequently, such as when a user is scrolling through a page and you only want to perform a specific action a maximum number of times per second.
Throttling is a technique for limiting the number of times a function can be called. After executing a function, all function calls will be suspended until a set period of time has passed.
The debounce function was one approach we initially considered, but throttle operates rather differently.
This and the debounce function both prevent unneeded actions from being fired. Let’s examine how it functions.
Upon the initial user click, an event is triggered. However, should the user click again during the predefined delay, subsequent events will not be generated.
Typically, when someone clicks, the event is activated. If you continue to click repeatedly, you will only receive a single click event every 10 seconds.
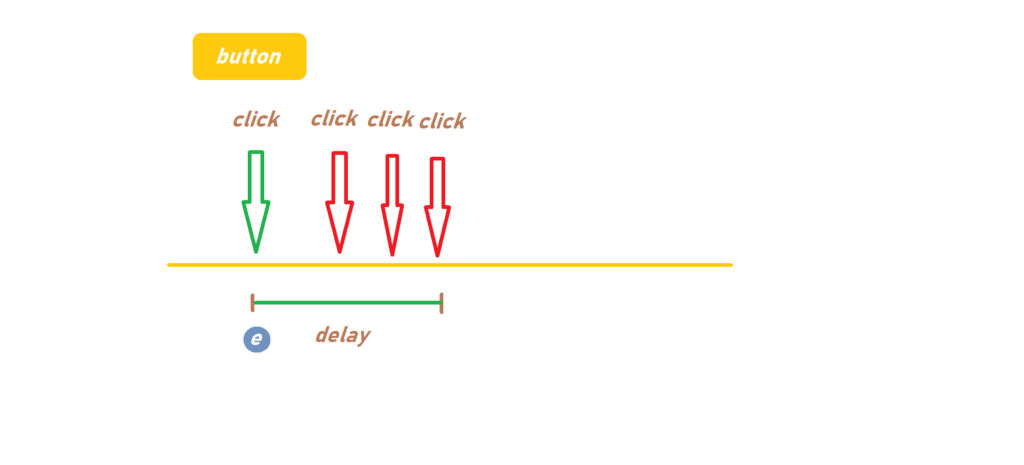
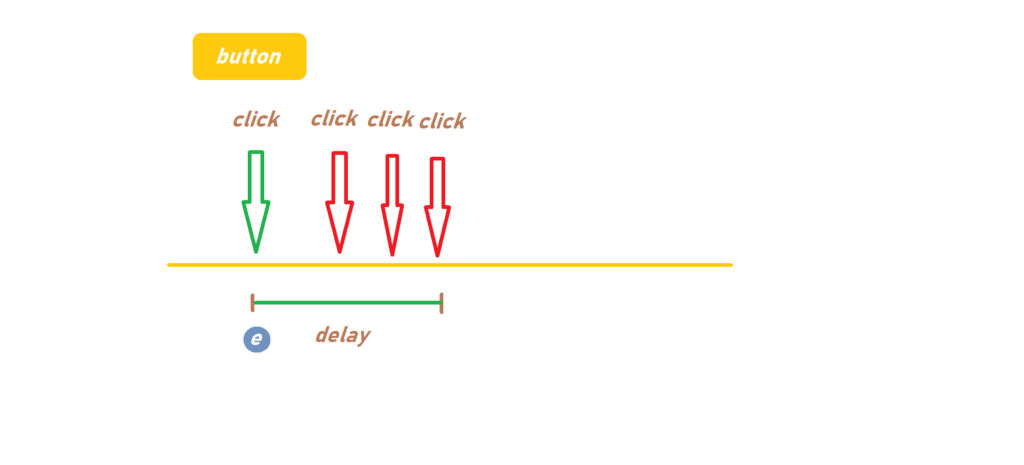
If you click multiple times in a quick succession
The event associated with that event would get fired multiple times and you need to avoid that.
const throttle = (fn,delay)=>{
let last = 0;
return function(...args){
const now = new Date().getTime();
if(now-last < delay){
return;
}
last = now;
return fn(...agrs);
}
}
document.getElementById("myid").addEventListner("click", throttle(e=>{
console.log('clicked');
},2000));
Compare to that if you look at debounce function, which looks little different to throttle.
In case of debounce function
It doesn’t really execute on a first click, basically, it delays the event as soon as the user clicks a second time.
If you keep clicking on a button over and over, nothing will get fired until the point where you actually stop.
Debounce vs Throttle
JavaScript Debouncing:
Debouncing offers a mechanism to postpone the execution of a function until after the most recent invocation, effectively consolidating multiple consecutive calls into a single one. This approach minimizes redundant executions.
It proves advantageous in scenarios where events occur at a high frequency, such as typing, scrolling, or resizing, and the intention is to perform an action only once the event ceases.
This technique proves particularly beneficial when you aim to aggregate sequential calls into one, to be executed after a specified time interval. For instance, consider a search bar where you wish to trigger a search function every time a user types. Rather than making a new request for each keystroke, you can wait until the user completes typing, e.g., 500 milliseconds after the last keystroke, before initiating the request.
JavaScript Throttling:
Throttling, on the other hand, provides a means to regulate the rate at which a function can be invoked. It restricts the number of times the function can be called within a defined time span.
Throttling proves useful in cases where an event fires too frequently, such as mouse movement, scrolling, or resizing, and there’s a need to curtail the frequency of function calls to enhance performance.
In contrast to debouncing, throttling serves well when the objective is to execute a function only a set number of times within a specific timeframe. For instance, imagine a scroll event handler where you want to update an element’s position on the screen as the user scrolls. However, you don’t want to update it with every pixel scrolled; instead, you aim to update it at intervals, perhaps every 200 milliseconds.
Both of these techniques can be implemented using various methods, including setTimeout, setInterval, requestAnimationFrame, and other browser APIs. Additionally, libraries like underscore and lodash offer their own implementations of debounce and throttle, which can be readily applied to any function.
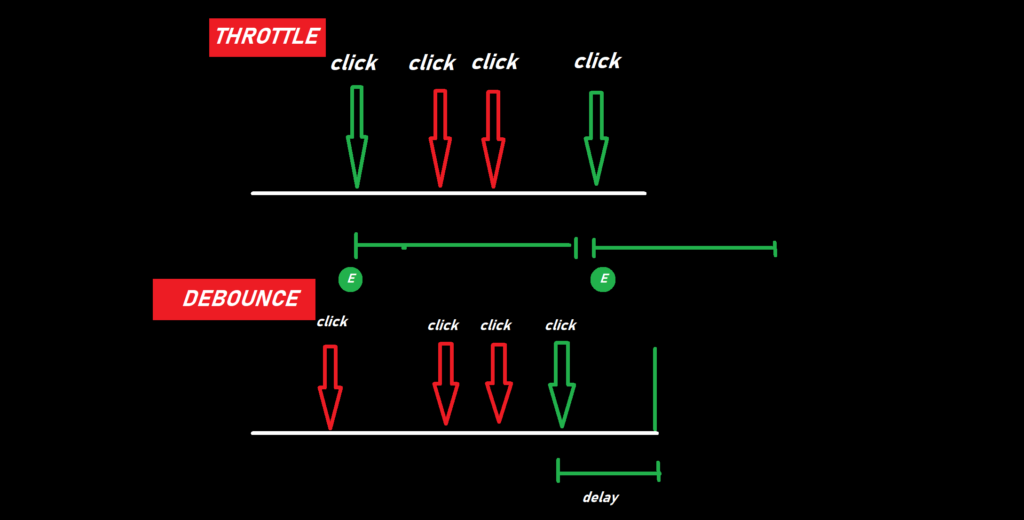
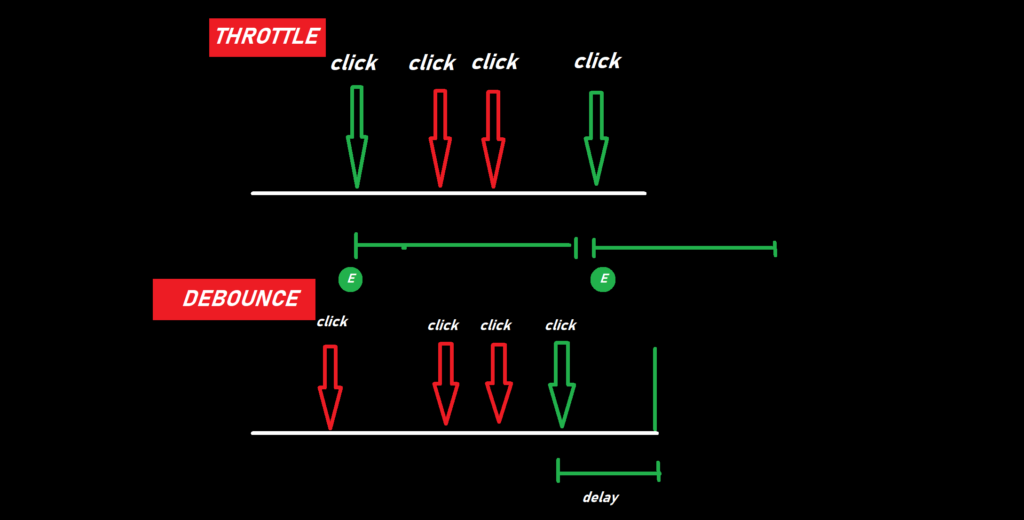
Furthermore, certain libraries such as RxJS and Lodash/Underscore offer their own built-in implementations of debounce and throttle. These implementations can be directly applied to any function you’re working with. This convenient feature enables you to seamlessly integrate debouncing and throttling into your code without the need for manual implementation, which can be complex in some situations.
Both of these techniques serve the common purpose of reducing unnecessary function calls and optimizing performance.
In JavaScript, debouncing and throttling differ primarily in their trigger mechanisms. Debouncing activates a function when a user remains inactive for a specified duration, while JavaScript throttling triggers a function at fixed intervals during user activity.
In debouncing, the initial click event is fired, whereas in throttling, the last click event is triggered after the predefined delay.
Debounce vs Throttle Example
Here’s a simple example to illustrate the difference between debouncing and throttling:
// Debouncing example
let debounceTimeout;
function debouncedFunction() {
console.log("Debounced function");
}
document.addEventListener("mousemove", function() {
clearTimeout(debounceTimeout);
debounceTimeout = setTimeout(debouncedFunction, 500);
});
// Throttling example
let throttleTimeout;
function throttledFunction() {
console.log("Throttled function");
throttleTimeout = null;
}
document.addEventListener("mousemove", function() {
if (!throttleTimeout) {
throttleTimeout = setTimeout(throttledFunction, 500);
}
});
In this illustration, we’re managing the mousemove event for the document. The debouncedFunction is invoked solely when the mouse ceases its movement for a continuous duration of 500 milliseconds, thanks to its debouncing mechanism.
Conversely, the throttledFunction is triggered at intervals of 500 milliseconds, unaffected by the frequency of mouse movements. This occurs because it operates under a throttling mechanism.
FAQs
What is throttling in JavaScript?
We frequently encounter a method referred to as throttling on websites, sometimes called the throttle function. In the context of throttling, the second click is not instantly executed; rather, it is carried out after a designated number of milliseconds or following the occurrence of the initial click.
What is debouncing in JavaScript?
By using the JavaScript debouncing approach, a function is only run after a predetermined interval without being called has elapsed. When a function is called quickly, such as when a user is entering into a text input field and you only want to take a certain action once they have finished typing, this can be helpful.
What is the difference between debounce time and throttle?
Debounce and throttle represent two commonly employed techniques in JavaScript to manage the pace at which events are handled.
Debounce: The debouncing method guarantees that a function is executed solely after a specific duration of inactivity, during which it has not been invoked. This technique proves valuable when the aim is to prevent a function from being triggered excessively, as seen when a user engages in text input. Debouncing introduces a delay in function execution until the user pauses their input, at which point it executes once.
Throttle: Throttling, on the other hand, ensures that a function can be executed no more than once within a designated time frame. It proves beneficial when there is a need to curtail the frequency of function calls, as observed when handling events like scrolling or resizing. Throttling immediately invokes the function and then suspends any subsequent calls until a predefined time interval has passed.What is debounce vs throttle vs delay?
While both are used to limit the frequency of a function’s execution, throttling causes execution to be delayed and lowers the number of notifications for an event that occurs repeatedly. Debouncing, on the other hand, combines a number of calls into a single call to a function, ensuring that multiple fires receive a single notification.
When should I use Debounce?
Passing a debounce as an argument to an event listener attached to an HTML element is the most typical application for one. Let’s look at an example to better comprehend what this looks like and why it is beneficial.
>>Also Read
Understanding of Bind, Call, Apply in JavaScript
JavaScript Prototypes and Inheritance